Create awesome offline-first apps that syncs
Keep writing client-side code. We provide the server.
Multi user access control
Sharded and scalable
Eager sync and collaboration when online
Periodic sync when app not active
Almost Zero Config
Create your database in the cloud by just running a simple npx command! Use the new dexie-cloud-addon to connect your Dexie database with the cloud and enjoy multi-user bidirectional sync.
Authentication
Passwordless email OTP is part of the service so you don't have to deal with setting up auth. Have auth already? Skip our default auth and use your own instead!
Access Control
A per-object access model lets your users keep their synced private data for themselves or shared with their teams or friends. Build your access control models using simple javascript objects.
Consistent Sync
Dexie Cloud doesn't just sync individual CRUD operations - it syncs the where-expressions along with the operations, making it possible to keep related data consistent.
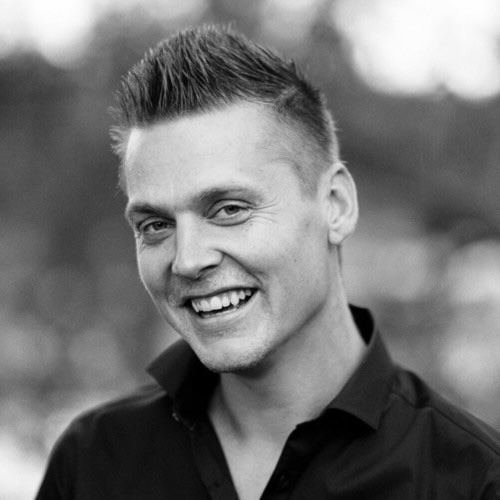
“After exploring several options, including major players like Firebase, I felt constrained by their complexity and locking structures. I sought a platform that not only fostered creativity but also expanded our possibilities.
That's when I discovered Dexie Cloud. In just one year, I've single-handedly built a hybrid web and mobile application for iOS and Android, complete with automatic data synchronization across all devices. The transition from traditional Dexie with live queries to fully integrated Dexie Cloud was seamless and took only a few minutes.
What truly sets Dexie Cloud apart is its ability to unleash productivity and nurturing creativity. Unlike other platforms, Dexie Cloud doesn't confine our visions; it expands them. It gives us the freedom to explore and experiment without being limited by complex structures or preconceived ideas.
Moreover, the support, documentation, and community have been invaluable. Dexie Cloud is more than just a service; it supports and inspires growth and progress.
I'm completely sold! Even considering alternatives like CouchDB or Firestore now feels restrictive and cumbersome. Dexie Cloud opens doors to a world of possibilities and makes me look forward to every step of our journey, including multiple apps, prototypes, and client projects."
Zenta AB is using Dexie Cloud to power their app To To-do. It's an AI-driven offline-first task manager that helps users plan their day and reach their goals: https://totodo.app
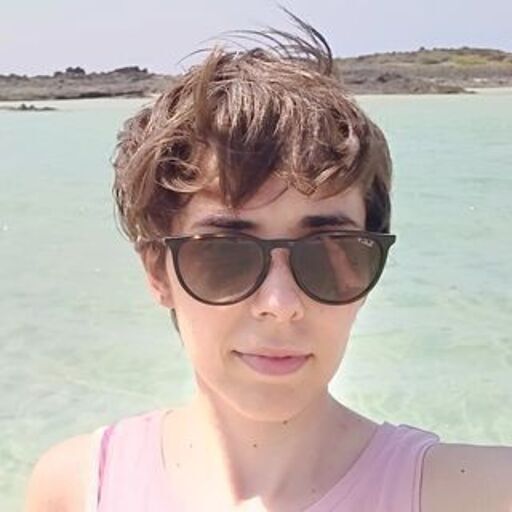
“I started using Dexie for my project, and I was worried about integrating user authentication and synchronization into it. However, when I discovered Dexie Cloud, I was amazed at how seamlessly I could integrate it into my app. Within minutes, I had my application fully synchronized and authenticated. The documentation provided by Dexie Cloud was also very helpful. It's well-organized and has lots of examples that made it easy to understand and use."
Routickr is an app that will help users to transform their goals into lasting habits: https://www.routickr.com.
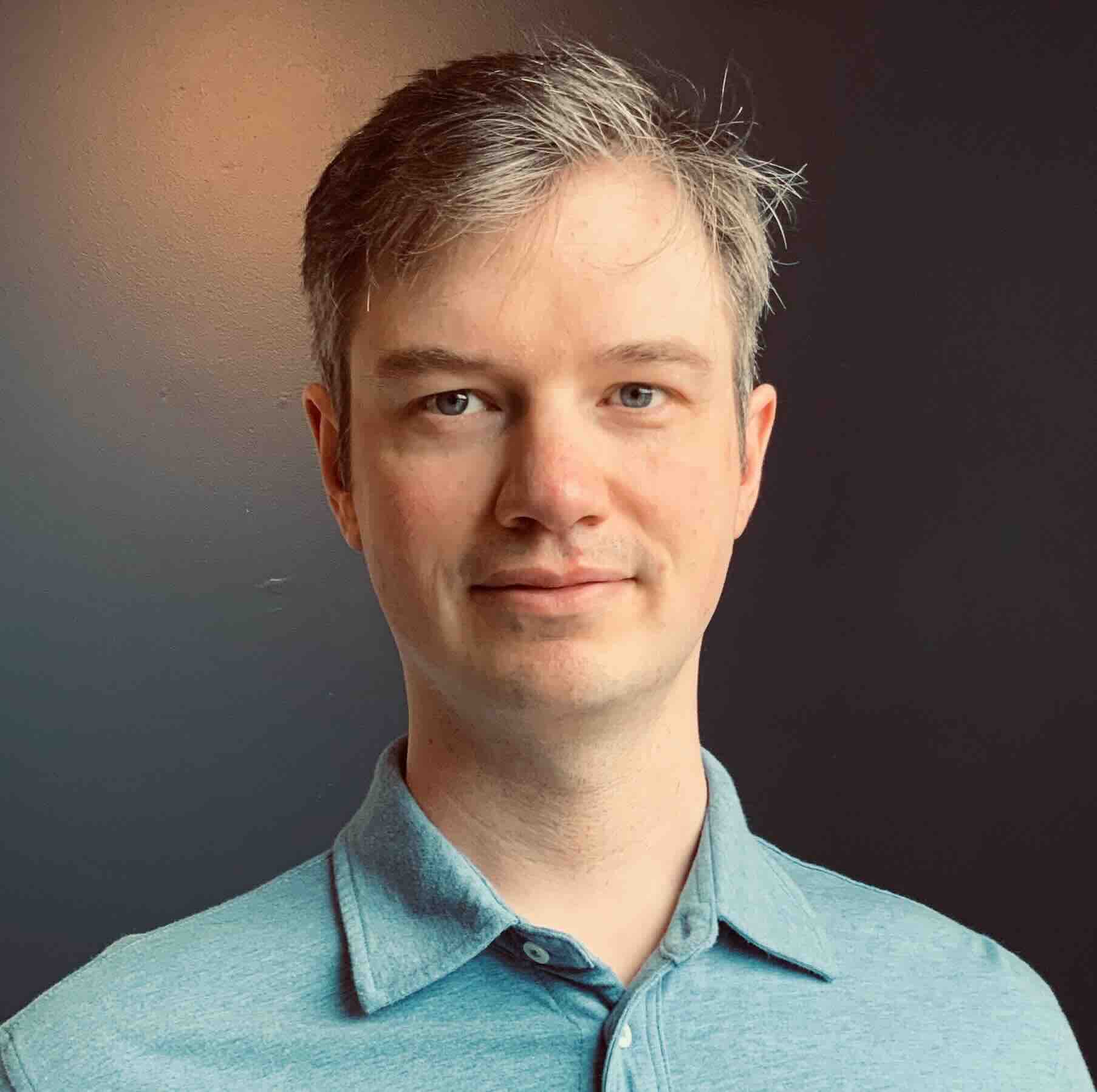
“Offline-first web apps are no longer difficult. Dexie Cloud gave Fablehenge a truly backendless experience and has been essential for our tiny dev team to ship big features like it already happened. It is the perfect abstraction in front of the endless complexity two-way sync normally entails. Just write your data to IndexedDB with Dexie's best-in-class API. It'll show up where it's supposed to. Nothing could be simpler."
Fablehenge is an app for story writers to help them track their character relationships, reorganize their outline, and manage their drafts: https://www.fablehenge.com/
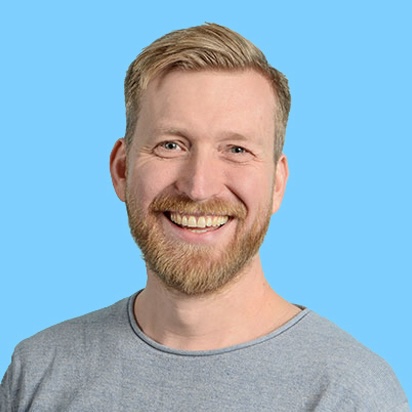
“The speed with which you can start and complete an observation is crucial to whether you will prefer my app to Notes - or even pen and paper - at all. The discovery of Dexie Cloud made me realize that my bird lists could be shared with others and that there were also ready-made code examples on how to manage invitations, rights and similar things.”
Birdlist is an app for birdwatchers that helps them log their observations, create lists, and share them with friends.
What it is:
- Think plain Dexie.js but also with online sync. Write completely Offline-First apps using Dexie.js as your sole storage model. Then let Dexie Cloud sync your data with the cloud.
- The data in the cloud is protected using authentication and authorization to prohibit unauthorized access.
- Data can be shared between multiple or individual users, making it possible to build reactive online collaboration apps hosted on a static web page without bothering about a server. And of course: with offline support so there's no problem sending a chat messages or look at the latest conversation while the network is down.
Get started
This guide assumes you're already using Dexie.js and now want to connect it to Dexie Cloud.
1. Create your database in the cloud
1. Create your db in the cloud
npx dexie-cloud create
You'll be prompted for email verification. Then the URL of your database will output to the console and stored in a new local file dexie-cloud.json. See the CLI docs.
2. Whitelist your app origin(s)
npx dexie-cloud whitelist http://localhost:3000
3. Upgrade dexie + install dexie-cloud-addon
npm install dexie@latest
npm install dexie-cloud-addon
4. Update your DB declaration
import Dexie from "dexie";
import dexieCloud from "dexie-cloud-addon";
const db = new Dexie('SyncedFriends', {addons: [dexieCloud]});
db.version(1).stores({
friends: '++id, name, age' // '++' = auto-incremented ID
friends: '@id, name, age' // '@' = auto-generated global ID
});
// Connect your dexie-cloud database:
db.cloud.configure({
databaseUrl: "https://<yourdatabase>.dexie.cloud",
requireAuth: true // optional
});
- The '@' character is optional and makes the primary key auto-generated unique string.
- databaseUrl is the one printed out in step 1.
5. Done!
Enjoy.
With this very simple and default setup, users will be requested to login using passwordless email OTP the very first time they visit your app from a device or browser. Learn how to customize authentication if you already have another authentication in place, or want to integrate with the authentication of your choice.
Use your db with the simple Dexie API you are used to for mutating and querying data. IDs marked '@' gets auto-generated keys similar to Dexie's ++ but with a globally unique string rather than an auto-incremented number.
Changes sync instantly with the cloud in both directions. When offline, changes are queued until next time user goes online. When online, changes will be synced eagerly in both directions. If user is idle for a time, the client will go over to periodic sync strategy instead of the eager sync. As soon as user starts interact with the application (mouse moves or key strokes), the app will go over to eager sync mode again.
Without taking advantage of the access control features, all data will be regarded private for the end user and stored on a per-user basis. Improve your app by allowing users to share things to their team or friends.
The Free Edition
Free (SaaS)
$ 0
- 3 prod users
- 100 MB storage
- Infinite eval users
- 10 connections
Choose this option to get started with Dexie Cloud
Enter the world of Dexie Cloud by building powerful apps that run in any browser, phone or desktop, superpowered with local-first storage.
All features are available including Authentication, Access Control, REST-API and Dexie Cloud Manager.
Continue developing your app as long as it takes! Its' forever free.
"I was procrastinating on adding Dexie Cloud as I was waiting until I had a decent block of time, but it took me all of 10 minutes."
Dexie Cloud Production
Production (SaaS)
$ 0.12 / user / mo
- 25+ prod users
- 25+ GB
- 50+ connections
- Infinite eval users
- Increased rate-limits
Choose this option when users get on-board
When it's time to get serious!
Subscription is purchased in packs of 25 seats for only $3 per month.
After purchase, use Dexie Cloud Manager to assign seats to your end users.
Increase or decrease number of seat packs with a few clicks in Dexie Cloud Manager.
Automate users and seat assignment using the REST api.
"Dexie Cloud gave Fablehenge a truly backendless experience and has been essential for our tiny dev team to ship big features like it already happened. It is the perfect abstraction in front of the endless complexity two-way sync normally entails. Just write your data to IndexedDB with Dexie's best-in-class API. It'll show up where it's supposed to. Nothing could be simpler."
On-Prem Silver
On-Prem Silver
$ 3,495
(one-time)
- Install on your own servers
- No limits or recurring costs
- 5 years software updates
- 1 year Silver Support
Opt for this when you need full control over your data.
Run the software run on your own servers or cloud infrastructure
License to use the software forever.
No limits on storage or number of users. No or recurring costs.
Gain complete control over the location of your data for legal or compliance reasons.
5 years of software updates and 1 year of Silver Support included in the price.
“After exploring several options, including major players like Firebase, I felt constrained by their complexity and locking structures. I sought a platform that not only fostered creativity but also expanded our possibilities."
On-Prem Gold
On-Prem Gold
$ 7,995
(one-time)
- Full Source code
- Install on your own servers
- No limits or recurring costs
- 5 years software updates
- 1 year Gold Support
When you need deeper integration and support
Buy off the software and do whatever you want with it (except competing with us)
License to use the software forever. Source code included.
No limits on storage or number of users. No or recurring costs.
Gain complete control over the location of your data for legal or compliance reasons.
5 years of software updates (binaries) and 1 year of Gold Support included in the price.
“I'm completely sold! Even considering alternatives like CouchDB or Firestore now feels restrictive and cumbersome. Dexie Cloud opens doors to a world of possibilities and makes me look forward to every step of our journey, including multiple apps, prototypes, and client projects."
Want to know more?
Examples
Create a role-based access controlled project management model
Integrate with PassportJS authentication